Getting started
Instant payments triggered from your UI
Introduction
HandCash allows developers to embed payments anywhere into your game.
Make your first payment
First, users must connect their wallet to your app to grant permissions and provide you with an authToken
.
This token allows you to access user profiles and make instant payments.
Follow the steps in this section to trigger your first payment using HandCash Connect.
Create your app in the dashboard
- Create a HandCash Developer account: https://dashboard.handcash.io
- Create a team.
- Create an app.
- Grab your
appId
andappSecret
required by the SDK. - Define your redirection URLs. Users will be redirected there when they authorize your app.
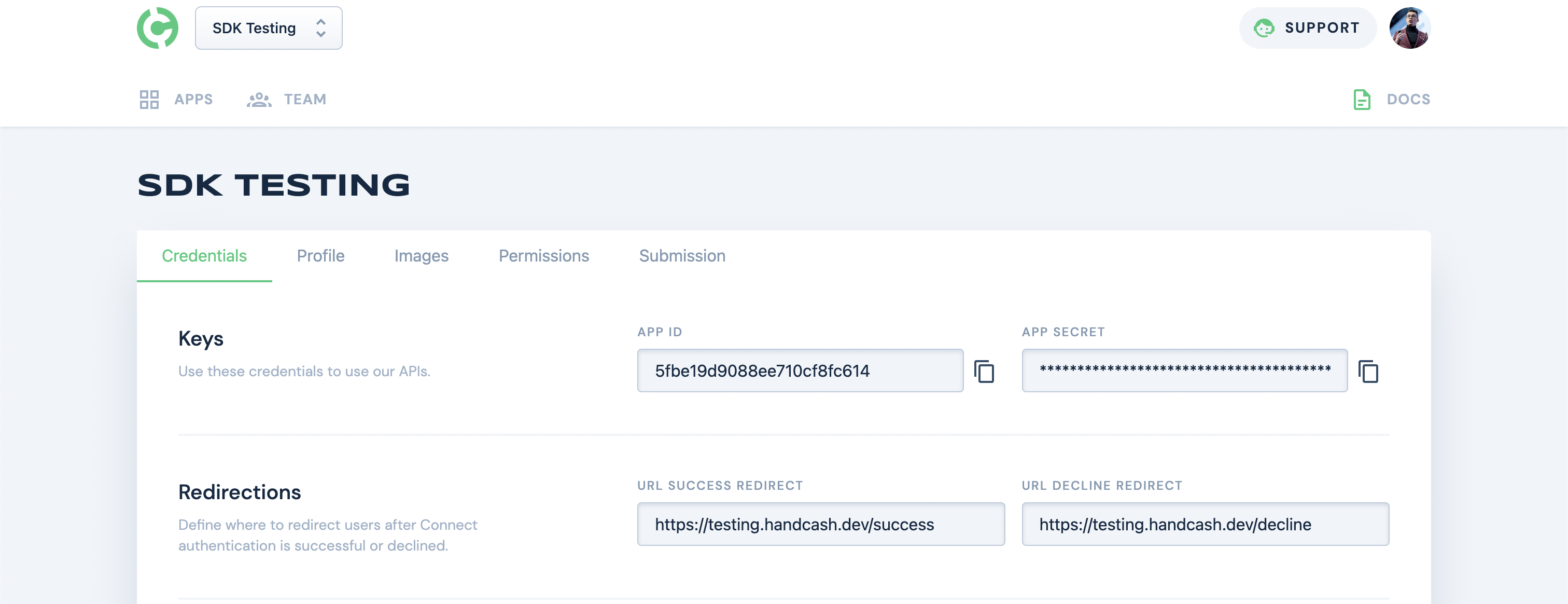
HandCash Dashboard preview
Install the SDK
Install the Connect SDK for Node:
npm i @handcash/handcash-connect
Import the module and initialize using the appId
and appSecret
:
const { HandCashConnect } = require('@handcash/handcash-connect');
const handCashConnect = new HandCashConnect({
appId: '<app-id>',
appSecret: '<secret>',
});
Redirect the user to HandCash to authorize your app
Generate the authorization URL and redirect the user to HandCash from your app.
const redirectionLoginUrl = handCashConnect.getRedirectionUrl();
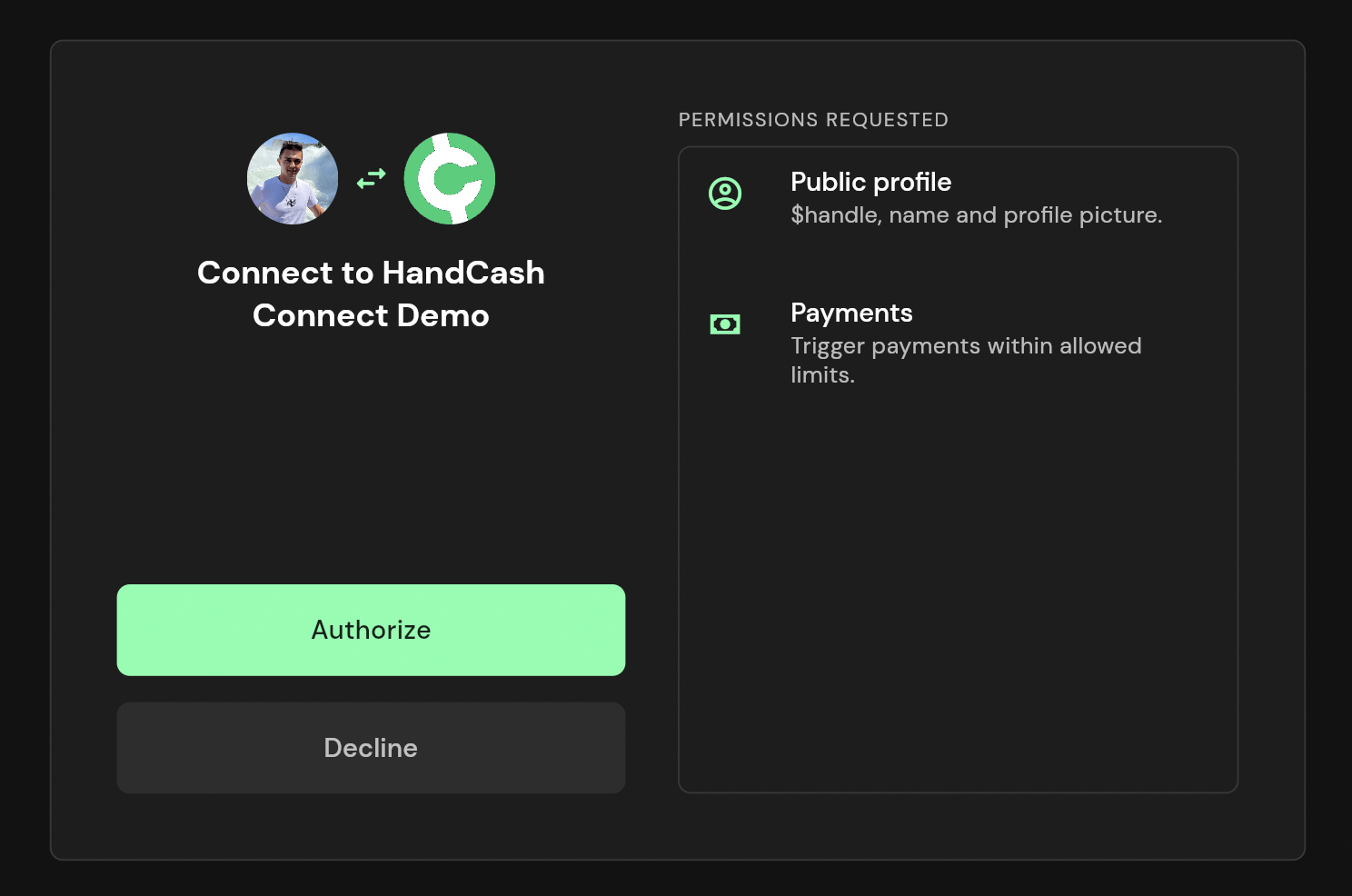
App authorization preview in the HandCash App
After the user has granted permissions to your app, they will be redirected to the authentication success URL you have set in the developer dashboard.
Initialize the user account
const account = handCashConnect.getAccountFromAuthToken(<authToken>);
Get the user profile
const { publicProfile } = await account.profile.getCurrentProfile();
console.log(`Hey $${publicProfile.handle}, welcome to my app!`);
Make a payment
const payParameters = {
payments: [{ destination: 'handcashteam', currencyCode: 'USD', sendAmount: 0.10 }]
};
await account.wallet.pay(payParameters);
Integrations
Updated 5 months ago